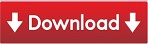
We want two images to “blend into” each other as one image. Image blending means combining two images with shared transparency. (Right) The same image resized to double its original size. Note the differences in their width and height axes instead.įigure 2: (Left) An image resized to half its original size. Both look the same because their aspect ratios are retained.
#Cv2 resize image free#
Feel free to be creative with the image dimension definitions. That creates an image of size (11126, 7418, 3). Img_RGB_bigger = cv2.resize(src=img_RGB, dsize=(width, height)) You can also make the image larger by multiplying its width and height by two, as follows: width = int(img_RGB.shape * 2) The output of the img_RGB_smaller.shape is (2781, 1854, 3), which is 50% smaller than its original size, (5563, 3709, 3).
#Cv2 resize image code#
The code shown takes the width and height values of the original image and divides them by two.
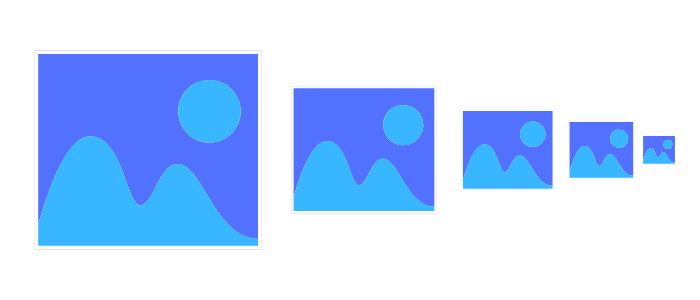
Yet, it is a good practice to use a scale factor to keep the original aspect ratio of the image.
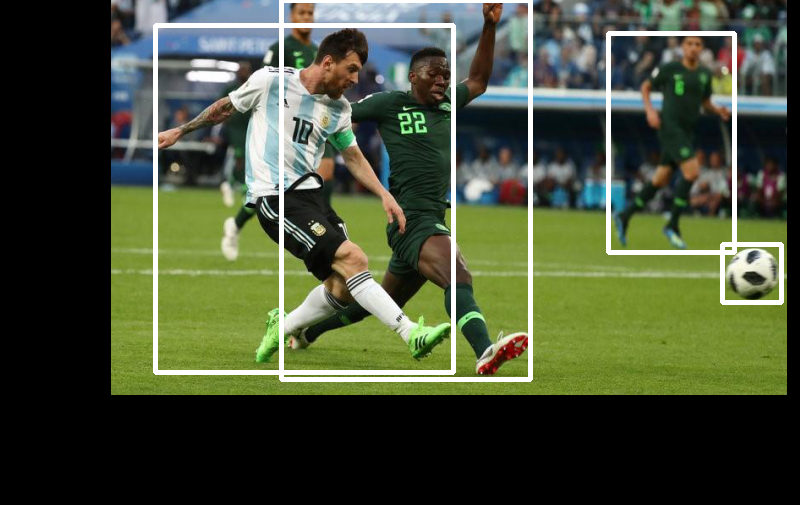
Note that you can supply any positive integer values to the dsize parameter of the resize method. Img_RGB_smaller = cv2.resize(src=img_RGB, dsize=(width, height))
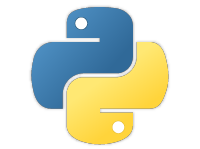
The following code resizes the image to be half its original size: width = int(img_RGB.shape / 2) It takes an image and an image dimension as parameters. Quiz time: which OpenCV method should you use to resize an image? You guessed it - the resize method. Make sure to pick the correct channels in operation. But using RGB images is not a requisite - feel free to use the BGR image if you prefer. We will use the RGB image as an example in the latter sections. The color-corrected image is shown on the right side of Figure 1. To fix this, we can use the OpenCV cvtColor method to convert the color channels from (B, G, R) to (R, G, B), as follows: img_RGB = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) Matplotlib displays the red channel as blue for the image on the left. (Right) The same image with (Red, Green, Blue) color channels.įigure 1 shows how different an image can look when its color channels are mixed up. To display an image, use the imshow method of the Matplotlib module as follows: plt.imshow(img)įigure 1: (Left) An image with (Blue, Green, Red) color channels. In case you ever wonder why your images look weird in OpenCV, it is that. But img_greyscale consists of only one channel (one color) while img has three.īy default, the imread method loads an image with a color order of blue, green, red. Both the variables have the same height and width values. The method returns information in the form of (height, width, channel). The shape method returns (5563, 3709) for the variable img_greyscale and (5563, 3709, 3) for img. Note that the images are loaded as NumPy arrays – one greyscale and another one in color. To load a greyscale image, we need to supply a second parameter of “0’” to the method: img_greyscale = cv2.imread('./photo.jpg', 0) By default, the method loads an image in color.
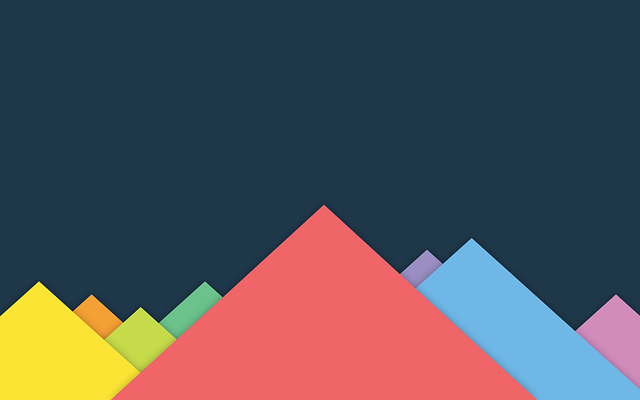
To load an image in the notebook, we use the imread method of the OpenCV module. It sets the backend of the Matplotlib module to display figures inline and not on a separate window.ĭone! Get your favorite photos ready - it’s time for experiments! Load Image and Convert Color Channels Note that the %matplotlib inline magic command is exclusive for Jupyter notebooks. Now execute the following lines of code in your notebook: import cv2 No surprise here - the installation should be straightforward and fast.
#Cv2 resize image install#
Then, install the mentioned modules in a Jupyter notebook: !pip install opencv-python Feel free to clone the GitHub repo of this tutorial.įirst, create a virtual environment for this project. Matplotlib is used to display images for comparing the “before and after”. NumPy is used to manipulate image arrays. For this tutorial, we need to install the OpenCV, NumPy, and Matplotlib modules.
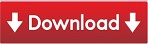